Cypress
Cypress is a framework for E2E testing and component testing.
To use Cypress in Modern.js, you need to install the dependencies first. You can run the following commands:
Next, create a cypress.config.ts
file and add the following content:
import { defineConfig } from 'cypress'
export default defineConfig({
e2e: {
setupNodeEvents(on, config) {},
},
})
Writing Test Cases
Now, use Cypress to write an E2E test case by first creating two Modern.js pages.
routes/page.tsx
import { Link } from '@modern-js/runtime/router';
const Index = () => (
<div>
<h1>Home</h1>
<Link to="/about">About</Link>
</div>
);
export default Index;
routes/about/page.tsx
import { Link } from '@modern-js/runtime/router';
const Index = () => (
<div>
<h1>About</h1>
<Link to="/">Home</Link>
</div>
);
export default Index;
Next, create the test case file:
cypress/e2e/app.cy.ts
describe('Navigation', () => {
it('should navigate to the about page', () => {
// Start from the index page
cy.visit('http://localhost:8080/')
// Find a link with an href attribute containing "about" and click it
cy.get('a[href*="about"]').click()
// The new url should include "/about"
cy.url().should('include', '/about')
// The new page should contain an h1 with "About"
cy.get('h1').contains('About')
})
})
The test file may lack type definitions for the API. You can refer to the Cypress - Typescript documentation to resolve this.
You can add the command to your package.json
:
package.json
{
"scripts": {
"test": "cypress open"
}
}
Run Test Cases
Execute the above test
command to run the test cases:
DevTools listening on ws://127.0.0.1:55203/devtools/browser/xxxxx
Cypress will open a headless browser. Following the prompts, you can find the corresponding test files and automatically run the E2E tests:
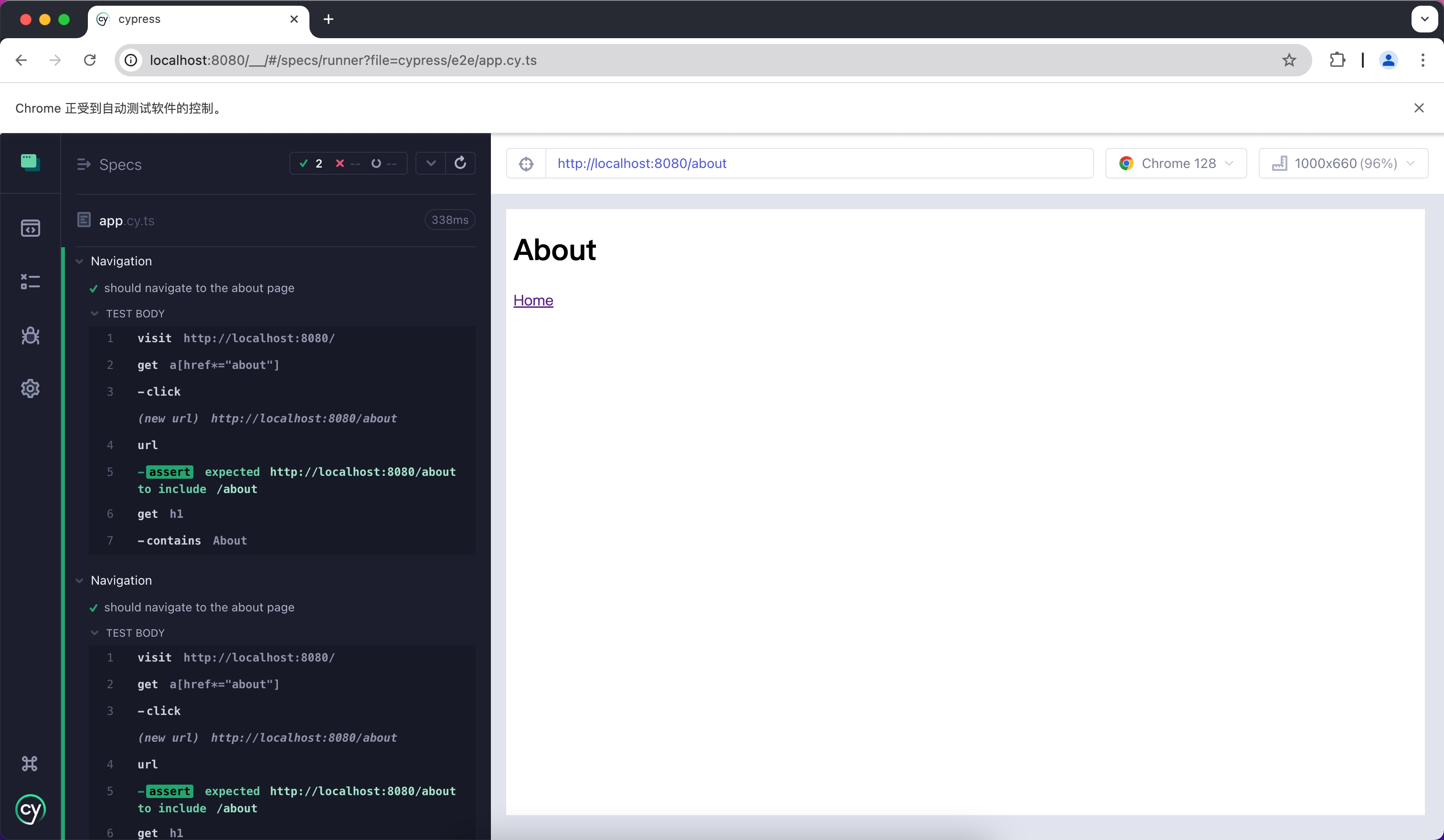